Model Views
It is often useful to be able to see views of the model without having to download and open the simulation file. You can define a set of model views that will be automatically captured at the end of the simulation.
coming soon!
There is extra functionality planned around Model views, this includes having views extracted at critical times related to results (e.g. the max/min value of a time history). Also, views can be extracted periodically during a simulation. More details are in the Roadmap
The ModelView attributes are not in lower case (as would be standard in python) because we
are lazily passing these to
ViewParameters. The
default view parameters are used for all parameters that are not defined in qalx_orcaflex
.data_models.ModelView
Defining views
There are 3 ways to define these:
Tags in General:
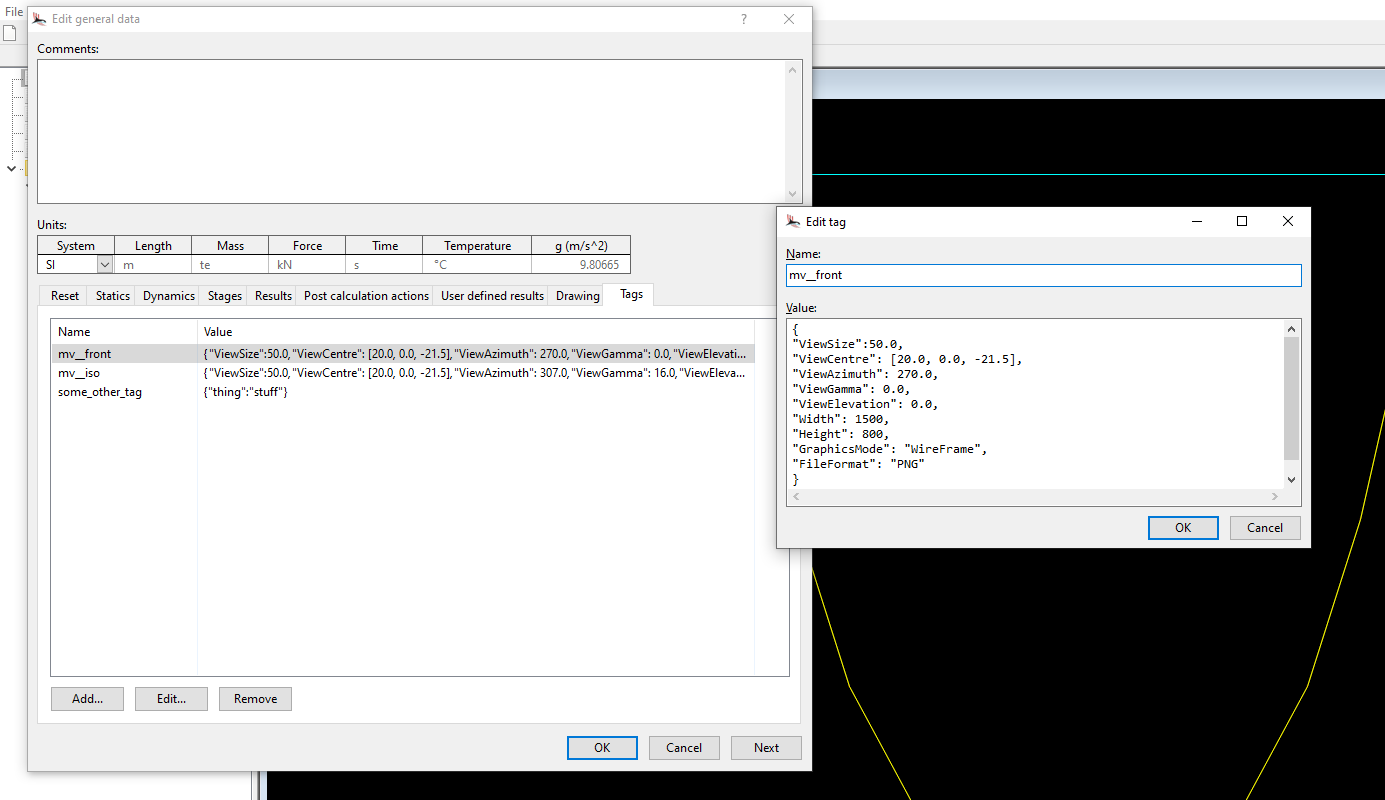
These are defined with a tag name that starts with mv and a double underscore (mv__). The text after the double underscore will be the name of the view. The value of the tag needs to be valid JSON.
Using data_models.ModelView
from qalx_orcaflex import data_models as dm
front_view = dm.ModelView(
ViewName="Front",
ViewSize=50.0,
ViewCentre= [20.0, 0.0, -21.5],
ViewAzimuth= 270.0,
ViewGamma= 0.0,
ViewElevation= 0.0,
Width= 1500,
Height= 800,
GraphicsMode= "WireFrame",
FileFormat= "PNG"
)
Copy paste the Edit view parameters form
Selecting all in the form (CTRL+A)
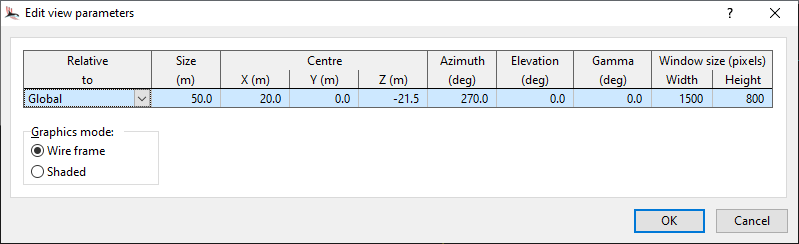
Will give you text like this:
Global 50 20 0 -21.5 270 0 0 1500 800
You can use the data_models.ModelView.***_from_form_str
class methods to build a data_models.ModelView
from this text like so:
from qalx_orcaflex import data_models as dm
front_view = dm.ModelView.wire_frame_from_form_str(
"Front",
"Global 50 20 0 -21.5 270 0 0 1500 800"
)
This will have exactly the same effect as the code in method 2 above.
Usage
We can add these model views to each model as it is added to the batch. This has the advantage of being able to extract different views for every simulation if required. The example below illustrates centering the view on the end of a line as it moves in each load case:
import OrcFxAPI as ofx
import qalx_orcaflex.data_models as dm
from qalx_orcaflex.core import ModelSource, OrcaFlexBatch, QalxOrcaFlex
qfx = QalxOrcaFlex()
batch_options = dm.BatchOptions(
batch_queue="example-batch-queue",
sim_queue="example-sim-queue",
)
with OrcaFlexBatch(name="My Hang-off Batch", session=qfx,
batch_options=batch_options) as batch:
m = ofx.Model()
line = m.CreateObject(ofx.otLine, "My Line")
for end_b_x in range(40, 80, 10):
line.EndBX = end_b_x
hang_off = 250 - end_b_x
hang_off_info = dm.RawInfo(
key="Hang-off point",
value=hang_off)
view = dm.ModelView(
ViewName=f"Front of hang-off @ {hang_off}m",
ViewSize=50.0,
ViewCentre=[end_b_x, 0.0, -21.5],
ViewAzimuth=270.0,
ViewGamma=0.0,
ViewElevation=0.0,
Width=1500,
Height=800,
GraphicsMode="WireFrame",
FileFormat="PNG"
)
current_speed = dm.ModelInfo(
object_name="Environment",
data_name="RefCurrentSpeed",
alias="Current Speed"
)
load_case_info = dm.LoadCaseInfo(
model_info=[current_speed],
raw_info=[hang_off_info])
batch.add(
ModelSource(m, f"HangOff={hang_off}"),
load_case_info=load_case_info,
model_views=[view]
)
These views can then be downloaded as per the below:
from qalx_orcaflex.core import QalxOrcaFlex
qfx = QalxOrcaFlex()
qfx.save_batch_views(
batch_name="My Hang-off Batch",
save_dir=r"C:/Users/AnneAlysis/OFX/Hang-offBatch/Views"
)
This will save the views as image files with the file name containing the case name and the name of the view:
