Welcome to qalx-OrcaFlex documentation!
qalx-OrcaFlex is a python package that contains tools for working with OrcaFlex batches on the qalx platform.
Installing
qalx-OrcaFlex is written in Python and can be installed via the Python Package Index (PyPi) with:
pip install qalx-orcaflex
Note
qalx-OrcaFlex requires Python >= 3.8
Warning
You will need to have a qalx API token configured. See the pyqalx docs.
Features
Below is a summary of the features, if you want to jump straight into using it then Quickstart is the place to go.
Batches
qalx-OrcaFlex gives you tools to build batches of OrcaFlex data files from various sources:
DirectorySource: adds all data files in a directory
ModelSource: adds an OrcFxAPI.Model object
FileSource: adds a single file as a source
DataFileItemsSource: adds existing qalx items (useful for re-running with updated post-processing data)
More details given in Building Batches.
Results
When adding data files, you can attach some required results to them which will be extracted automatically when the simulation is complete. The results will also be summarised for each batch.
These can be added in two main ways, either by building results objects in python:
from qalx_orcaflex import data_models as dm
range_graph = dm.RangeGraph(
object = "Line",
variable = "Effective Tension",
period = data_models.Period(
from_time=0,
to_time=10
)
)
time_history = dm.TimeHistory(
object= "Line"
variable = "Bend Moment"
period = dm.Period(
stage_number= 1
)
object_extra=dm.ObjectExtra(
line_named_point= "End A"
)
)
required_results = (range_graph, time_history)
or you can write a yaml file:
Results:
- RangeGraph:
object: Line
variable: Effective Tension
period:
from_time: 0
to_time: 10
- TimeHistory:
object: Line
variable: Bend Moment
period:
stage_number: 1
object_extra:
line_named_point: End A
These are then added to the batch with the data file.
For more details see Results.
Load case information
Results in a batch can be linked to information about the load case. Sometimes this is data that is contained in the model e.g. RefCurrentSpeed. And sometimes it is information that represents many data items e.g. “Far condition”, “100yr Wave”.
You can add either of these types of information to each job in a batch:
from qalx_orcaflex import data_models as dm
current_speed = dm.ModelInfo(
object_name = "Environment",
data_name = "RefCurrentSpeed",
alias = "Current Speed"
)
ret_period = dm.RawInfo(
key = "Wave return period"
value = "100yr"
)
offset = dm.RawInfo(
key = "Vessel offset"
value = "Far"
)
load_case_info = dm.LoadCaseInfo(
model_info=[current_speed],
raw_info=[ret_period, offset])
For more details see Load Case Information.
Model views
It is often useful to be able to see views of the model without having to download and open the simulation file. You can define a set of model views that will be automatically captured at the end of the simulation.
Attention
The ModelView attributes are not in lower case (as would be standard in python) because we are lazily passing these to ViewParameters
There are 3 ways to define these:
Tags in General
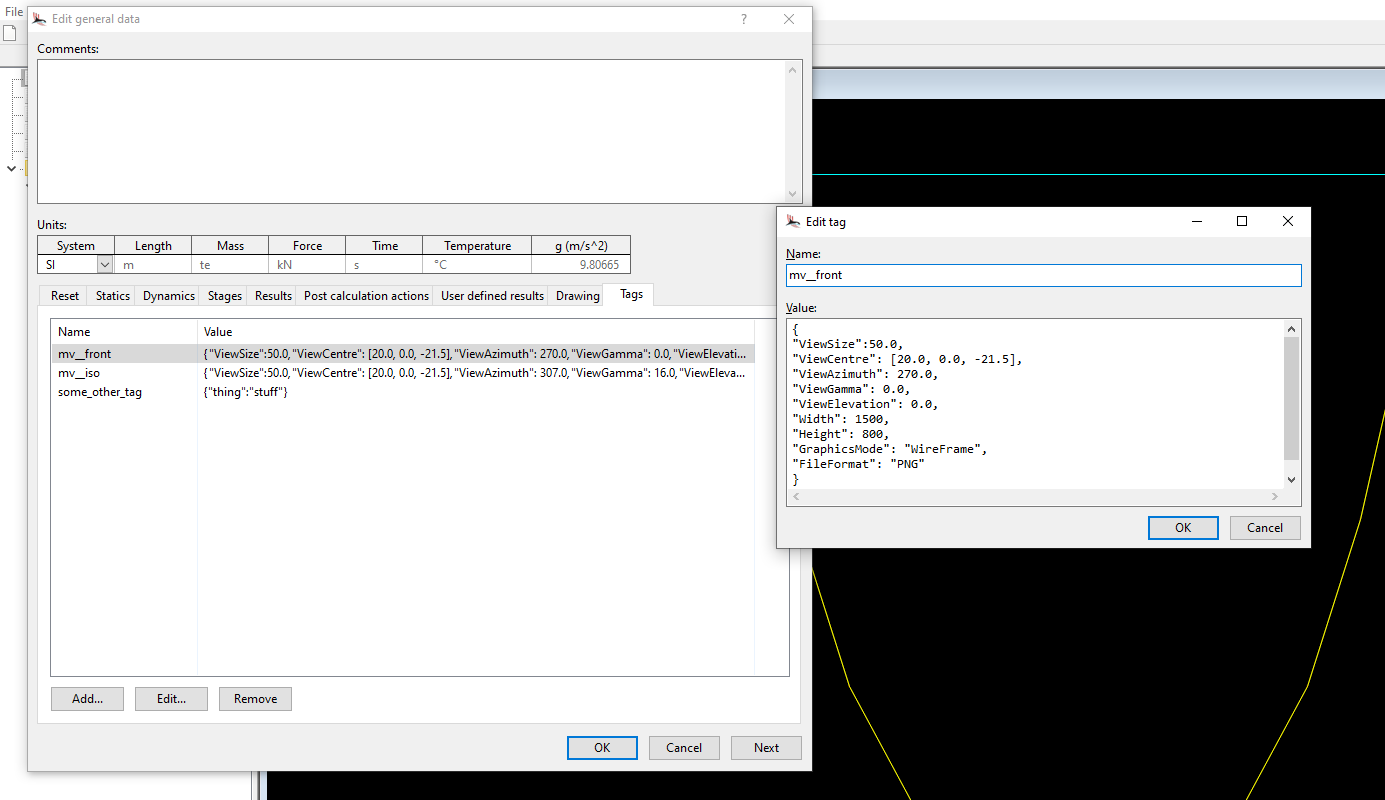
These are defined with a tag name that starts with mv and a double underscore (mv__). The text after the double underscore will be the name of the view.
Using data_models.ModelView
from qalx_orcaflex import data_models as dm
front_view = dm.ModelView(
ViewName="Front",
ViewSize=50.0,
ViewCentre= [20.0, 0.0, -21.5],
ViewAzimuth= 270.0,
ViewGamma= 0.0,
ViewElevation= 0.0,
Width= 1500,
Height= 800,
GraphicsMode= "WireFrame",
FileFormat= "PNG"
)
Copy paste the Edit view parameters form
Selecting all in the form (CTRL+A)
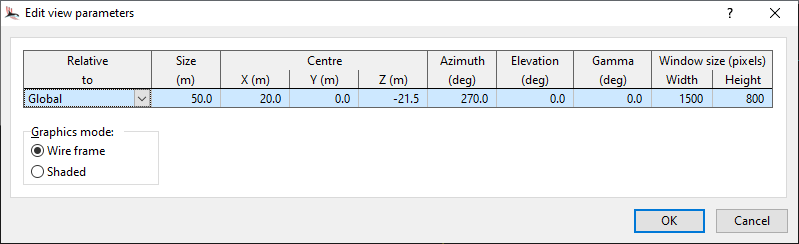
Will give you text like this:
Global 50 20 0 -21.5 270 0 0 1500 800
You can use the data_models.ModelView.***_from_form_str
class methods to build a data_models.ModelView
from this text like so:
from qalx_orcaflex import data_models as dm
front_view = dm.ModelView.wire_frame_from_form_str(
"Front",
"Global 50 20 0 -21.5 270 0 0 1500 800"
)
This will have exactly the same effect as the code in method 2 above.
More details are available in Model Views.
Smart statics
One of the limitations of building batches of models that are run automatically is that for complex models, especially those that implement line contact data, changes to the model data can result in a model that solved in statics in the base model failing to solve in statics in the load case model.
Smart statics helps overcome these issues by allowing you to add object tags that will be used to iteratively find a model that solves in statics. There are 4 types of adjustment that can be specified:
absolute: set to a random absolute value between limits
nudge: changed incrementally on each attempt
cycle: set by cycling through all choices in turn
choice: set to a random selection of choices
Tags need to be defined on the object that you want to adjust and start with “ss” and a double underscore (ss__). The text after the underscores should be the data name that needs adjusting (and another double underscore and the index if required). Some examples:
Moving the free EndB of a line around makes it solve:
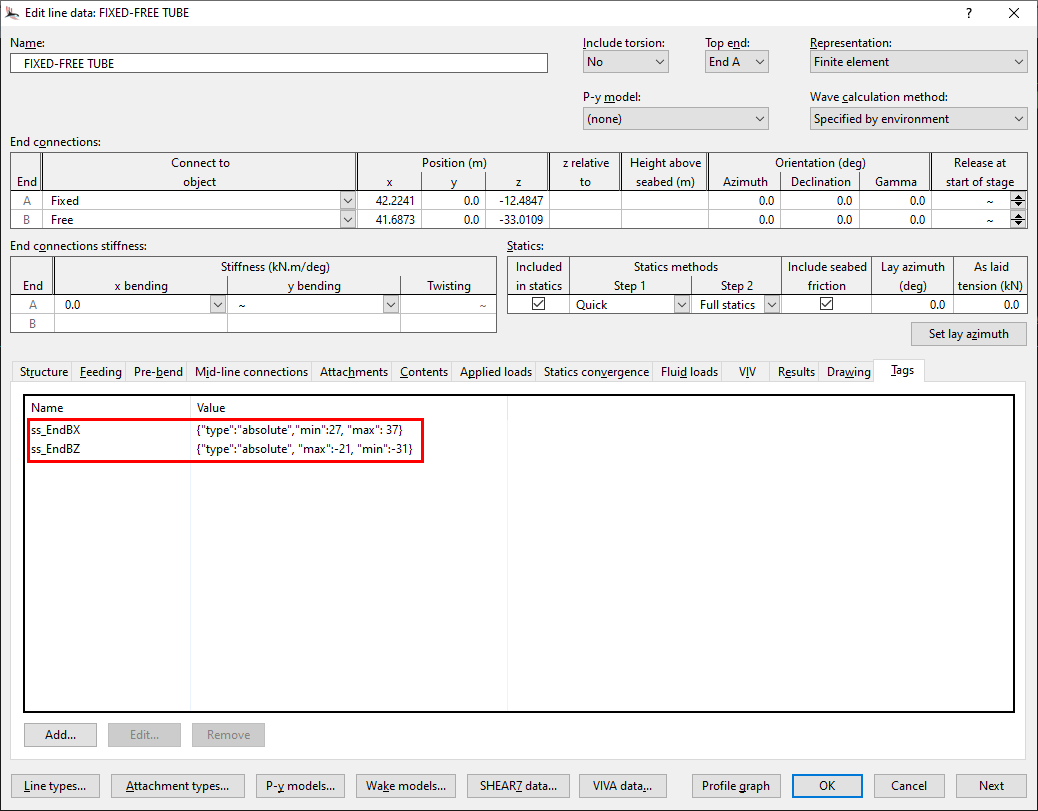
Changing the Step 1 statics method makes it solve:
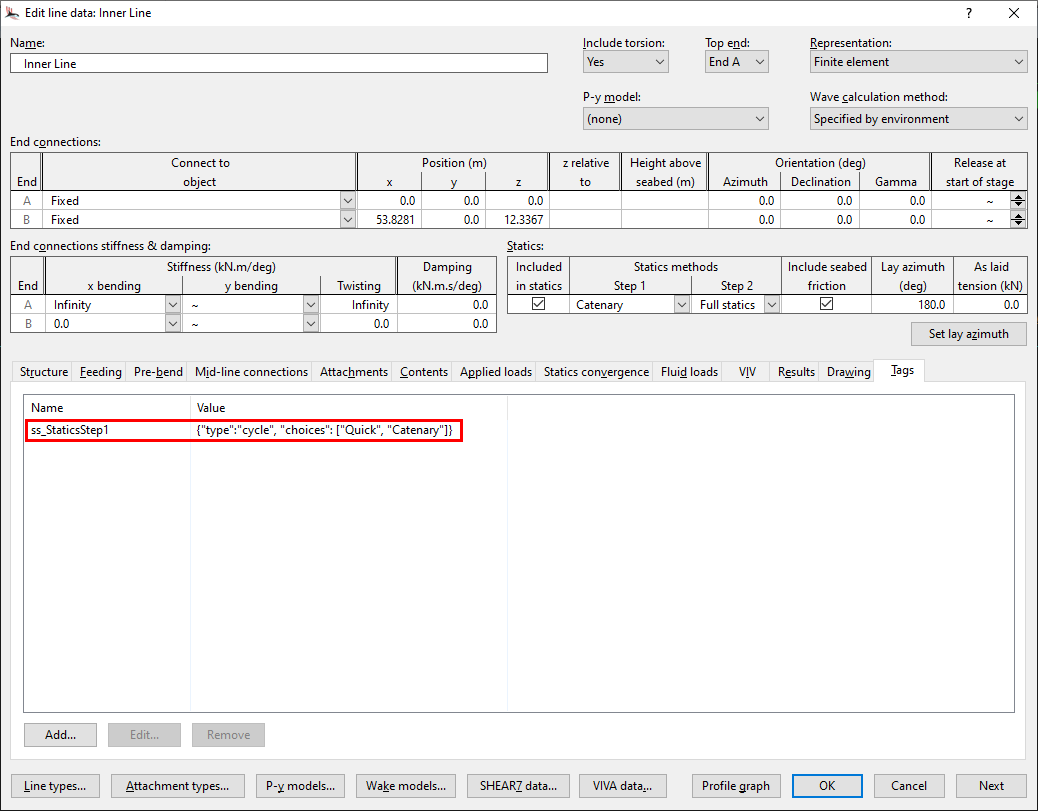
Changing the damping on a line makes it solve:
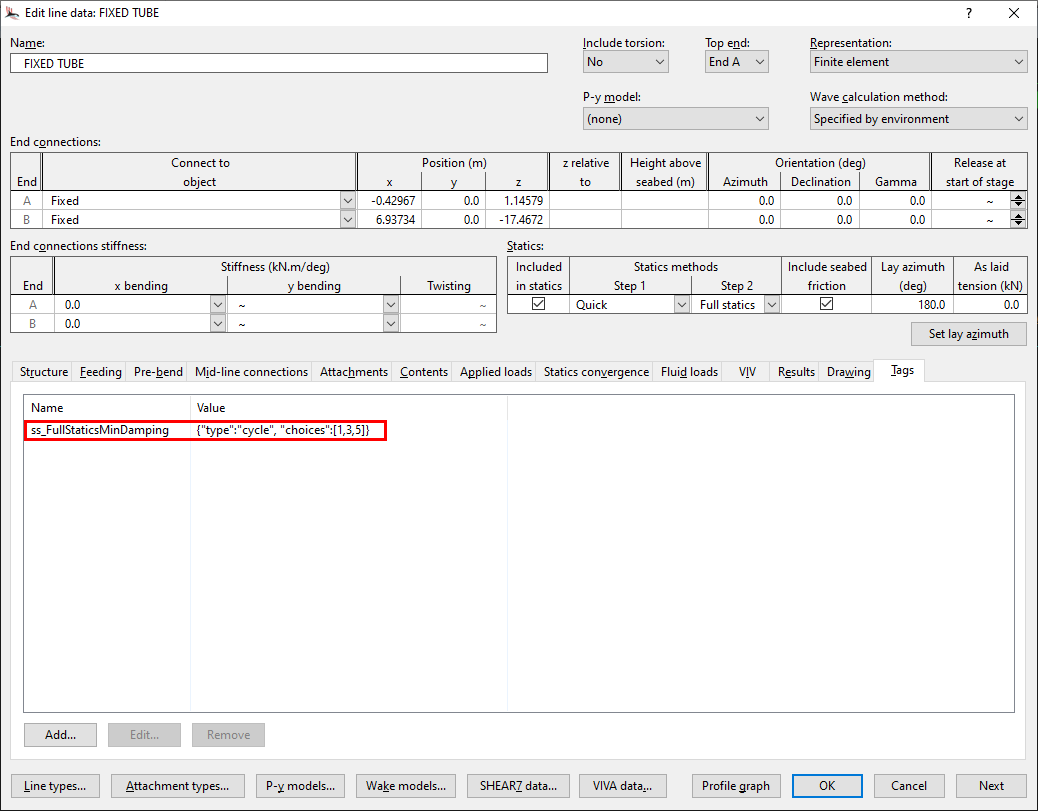
Increasing the whole system minimum damping makes it solve:
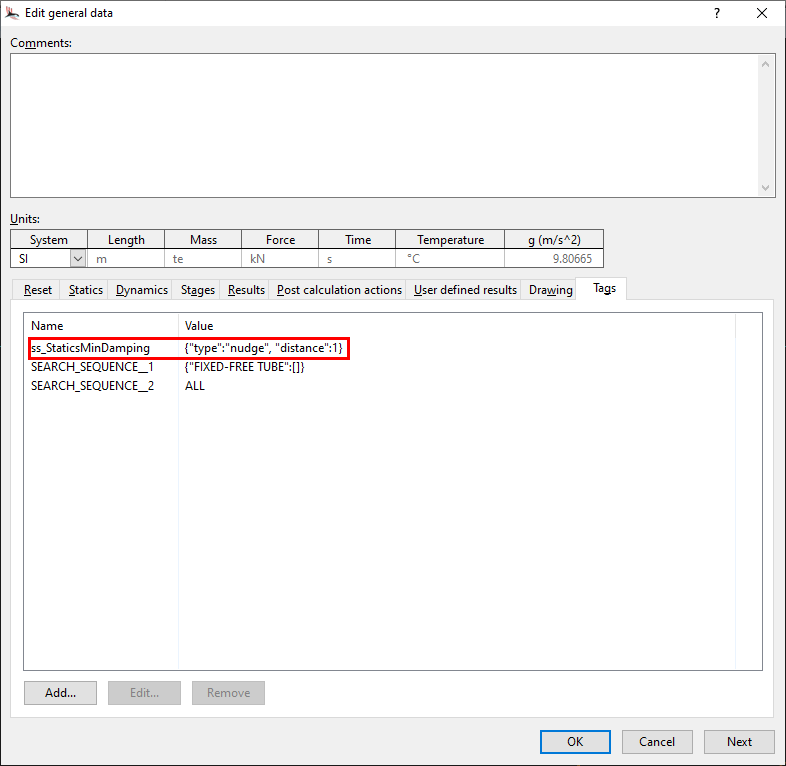
By default, on each iteration, all the adjustments will be attempted. So if you have specified 1-4 above then on each iteration smart statics will change the data on all the objects. However, sometimes this isn’t what you want to happen. To help with this, you can specify a SEARCH_SEQUENCE__# tag in General:
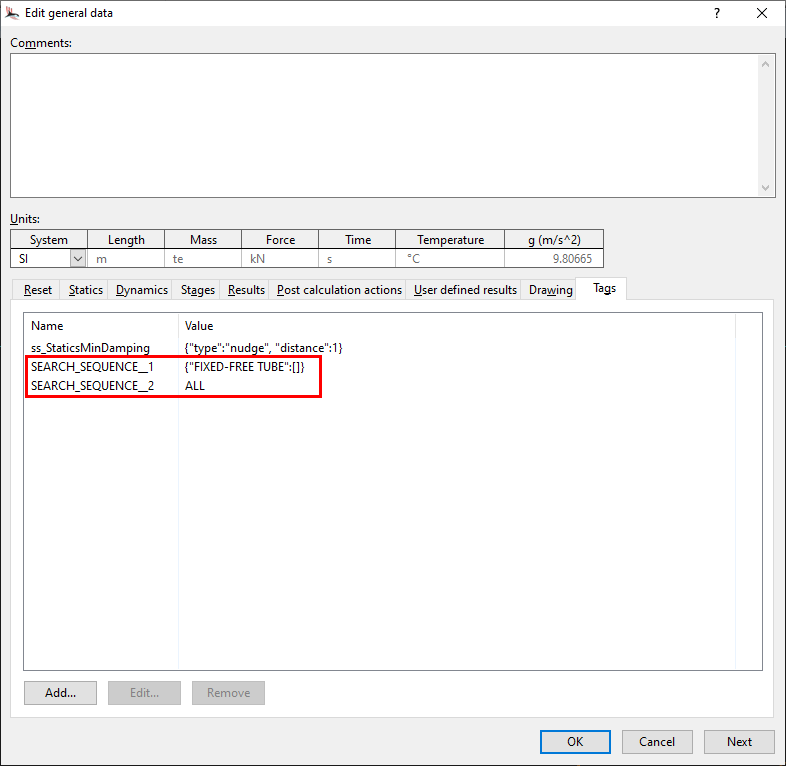
The above example will first attempt to get a solution by only changing all data items on “FIXED-FREE TUBE” if this is not successful then it will try to adjust all the items.
More details are available in Smart Statics.
Contents:
- Quickstart
- Building Batches
- Batch Management GUI
- Results
- Load Case Information
- Model Views
- Model Videos
- Smart Statics
- Bots
- Factory Plans
- Custom Bots
- Custom Interfaces
- Hints and tips
- API Documentation
- Roadmap
- Better API for dealing with completed batches (id:4013)
- A command line interface (id:4014)
- Custom results specification (id:4015)
- Linked Statistics and Extreme Statistics (id:4016)
- Model views at key result points (id:4017)
- Results summary spreadsheet automatically created (id:4019)
- Optional progress model views (id:4020)
- Automatic batch cancellation on result breach (id:4021)
- Allowed to define “Model deltas” from a base model (id:4022)
- Option to extract all model data into qalx (id:4023)
- Changelog
- Licenses